In C programming, variables are containers that hold data values. Each variable in C has a specific type, such as int
, float
, or char
, which determines the type of data it can hold and the amount of memory it occupies. By using variables, we can perform calculations, store intermediate results, and manipulate data throughout our programs.
In this article, we’ll explore the concept of variables, their types, syntax, and how they’re declared, initialized, and used in C programs.
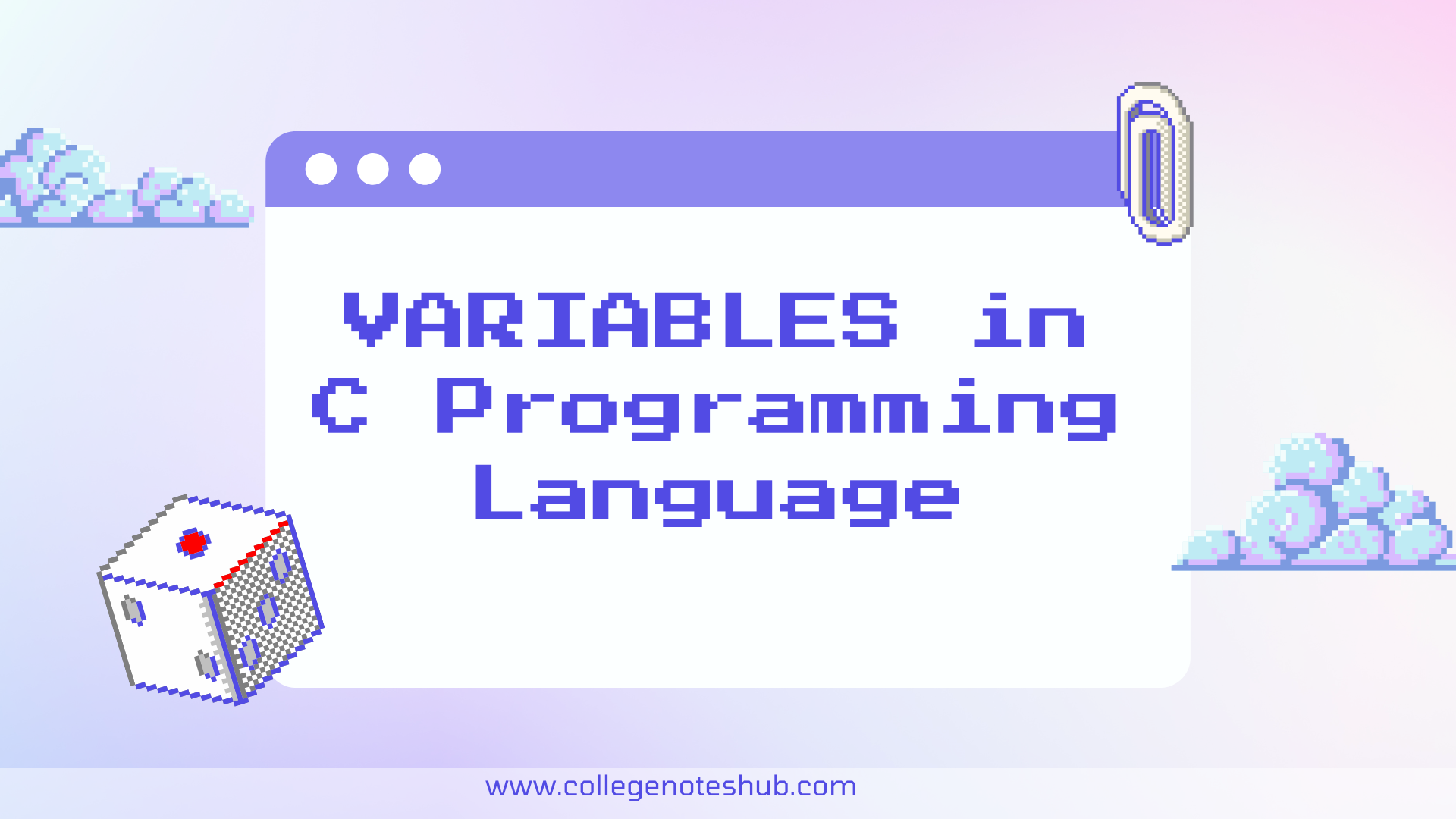
Table of Contents
What are Variables in C?
Variables are a foundational concept in any programming language, including C. They act as named storage locations in memory, allowing us to store and manipulate data. In C, variables are essential because they enable programmers to work with data effectively and make code readable and manageable.
Declaring and Initializing Variables in C
To use a variable in C, we first need to declare it, specifying its data type and name. Optionally, we can also initialize it by assigning an initial value at the time of declaration.
Syntax for Declaring Variables
data_type variable_name;
C- data_type: Defines the type of data the variable will store (e.g.,
int
,float
,char
). - variable_name: A unique identifier for the variable.
Syntax for Initializing Variables
data_type variable_name = value;
CExamples of Variable Declaration and Initialization
Example 1: Integer Variable
#include <stdio.h>
int main() {
int age; // Declaration
age = 21; // Initialization
printf("Age: %d\n", age);
return 0;
}
CExplanation:
In this example, we declare an integer variable age
and initialize it with the value 21
. The printf
function is then used to display the value of age
.
Example 2: Float Variable
#include <stdio.h>
int main() {
float height = 5.9; // Declaration and Initialization
printf("Height: %.1f\n", height);
return 0;
}
CExplanation:
Here, we declare a float variable height
and initialize it with the value 5.9
. The .1f
format specifier in printf
limits the output to one decimal place.
Example 3: Character Variable
#include <stdio.h>
int main() {
char grade = 'A'; // Declaration and Initialization
printf("Grade: %c\n", grade);
return 0;
}
CExplanation:
We declare a character variable grade
and initialize it with the value 'A'
. The %c
format specifier is used to print a single character.
Rules for Naming Variables in C
- Begin with a Letter: Variable names must start with a letter (A–Z or a–z) or an underscore (
_
). - No Special Characters: Special characters other than underscore are not allowed.
- Case-Sensitive: C is case-sensitive, meaning
age
andAge
are different variables. - Avoid Keywords: Reserved keywords in C, like
int
andfloat
, cannot be used as variable names.
Types of Variables in C
C provides different types of variables based on their scope and lifetime:
- Local Variables: Declared inside a function and only accessible within that function.
- Global Variables: Declared outside any function and accessible throughout the program.
- Static Variables: Retain their value between function calls.
- Automatic (auto) Variables: Default variable type inside functions, which gets created and destroyed with the function.
- External (extern) Variables: Declared in one file and can be used in another file.
Example: Local and Global Variables
#include <stdio.h>
int globalVar = 10; // Global variable
int main() {
int localVar = 20; // Local variable
printf("Global Variable: %d\n", globalVar);
printf("Local Variable: %d\n", localVar);
return 0;
}
CExplanation:
In this example, globalVar
is a global variable accessible throughout the program, while localVar
is a local variable limited to the main
function.
Variables in C are essential for storing and manipulating data. By understanding how to declare, initialize, and use different types of variables, programmers can write more efficient and organized code. In addition, knowledge of variable scope and lifetime allows for better control of data flow within programs. As you proceed with C programming, mastering variable usage will be a crucial step in building effective and readable programs.
By experimenting with variables and understanding their types and rules, you’ll be well on your way to creating powerful C programs.