Before starting the journey of Python Programming Language, let us learn What a Programming Language mean? A Programming Language is a set of instructions / language that humans use to communicate with computers. It consists of a set of rules, syntax and vocabulary that developers use to build software programs, applications and control systems. It help humans for instructing computers to perform specific tasks like calculations, data processing, automation and much more.
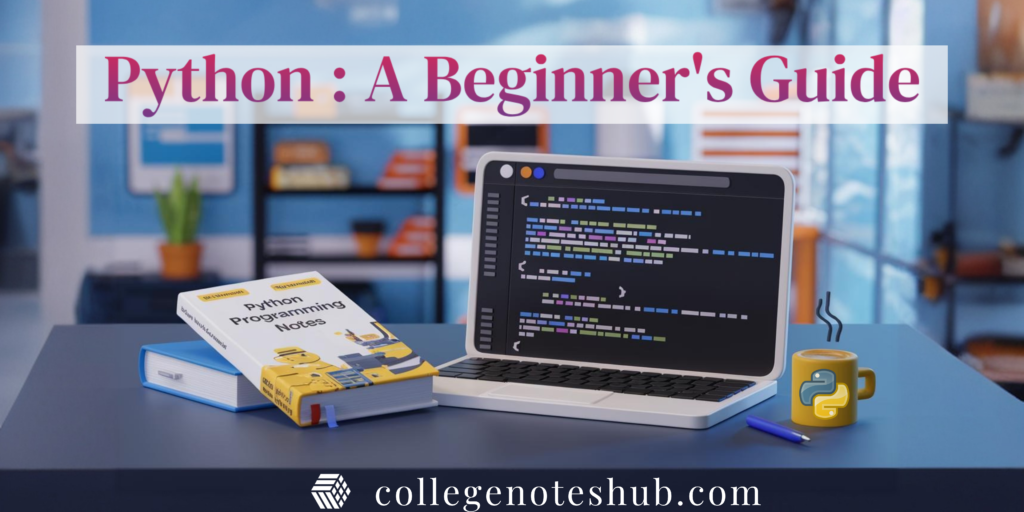
Table of Contents
Definition & How It Looks
Python is a high-level, powerful, interpreted programming language widely used for its simplicity, readability and versatility. It was mainly developed for emphasis on code readability and its syntax allows programmers to express concepts in fewer lines of code.
- A Glimpse into Clean and Powerful Code – PYTHON.
# This is a comment explaining what the code does
print("Hello, World!") # Prints "Hello, World!" to the console
Python- Explanation : Simple Python Program which prints a greeting message to the console.
#
is used for explaining the code which are known as Comments, these are ignored during execution.print()
is a built-in function to display text, variables, etc….
History of Python
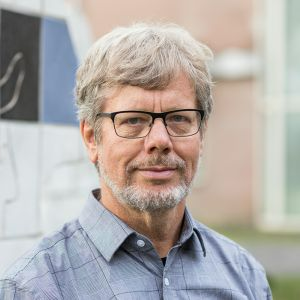
The History of Python begins in the late 1980s when Guido van Rossum, a Dutch programmer, started developing a new programming language after getting Inspired by the ABC programming language. His mission was to create a powerful and easy to read language. In December 1989, Guido started implementing Python at the Centrum Wiskunde & Informatica (CWI) in the Netherlands and was further developed by Python Software Foundation. The name “Python” was inspired by the British comedy series Monty Python’s Flying Circus, which van Rossum enjoyed. Python was initially designed as an alternative to ABC, capable of exception handling and interfacing with the Amoeba operating system.
- Key Milestones in Python’s History:
- 1991 : Python 0.9.0 was released, calling the first public release of the language. This version included core features such as exception handling, functions, and the math module (previously named as mod module).
- 1994 : Python 1.0 was released, introducing significant features like functional programming tools (for e.g reduce, filter, map, lambda) & support for modules, exceptions, and basic data types like lists and dictionaries.
- 2000 : Python 2.0 was released, with additional features like garbage collection list comprehensions. This version of python became very Popular and was continued for nearly 20 years, receiving updates and improvements over time. However, it had limitations and certain design decisions which made it challenging to expand without breaking compatibility.
- 2008 : Python 3.0 was released, introducing major changes to the language for resolving some of its fundamental design flaws. Python 3.0 was not fully backward-compatible with previous versions due to its new featues like modifying the print statement to a function and revising string handling to better support Unicode.
- 2020 : Python 2.7.18 was the last release of Python 2, marking the end of its official support. Thereafter, Python 3 became the Standard with Continuous updates like asynchronous programming, type hinting and performance improvements.
Why Python? Its Unique Features and Benefits
1. Simple and Readable Code Structure – Python’s syntax emphasizes readability. It uses indentation instead of braces to define code blocks which makes code more simple, readable and easy to modify, especially for beginners.
- Example :
def greet(name):
print(f"Hello, {name}!") # Simple, human-readable syntax
greet("Alice") # Output: Hello, Alice!
Python2. Interpreted Language – Python code runs line by line rather than being compiled everything at a time. This enables interactive testing and faster debugging, as you can find out errors and results immediately. It helps in Prototyping as we can run each line of code individually in a Python Shell.
- Example :
x = 5 + 10
print(x) # Output: 15
Python3. Dynamic Typing – Python is dynamically typed, so you don’t need to declare variable types explicitly. This makes coding more faster and flexible.
- Example :
age = 25 # age is an integer
age = "twenty-five" # Now age is a string
print(age) # Output: twenty-five
Python4. Object-Oriented and Procedural Programming Support – Python supports both object-oriented programming (OOP), in which data and functions are encapsulated within objects, and procedural programming, in which the functions exist independently of objects. This flexibility is useful for wide range of applications.
- OOP Example :
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print(f"{self.name} makes a sound")
dog = Animal("Dog")
dog.speak() # Output: Dog makes a sound
Python- Procedural Example :
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Output: Hello, Alice!
Python5. Extensive Standard Library – Python adopts a “batteries-included” philosophy, offering an extensive standard library designed for managing various tasks, including string operations, file handling, data manipulation and additional functions. This standard library facilitates developers in executing routine tasks without the need for external package installations.
- Example :
from datetime import datetime
current_time = datetime.now()
print("Current Date and Time:", current_time)
Python6. Rich Ecosystem of Third-Party Libraries – Python’s incredible ecosystem is full of libraries for data science, web development, automation, machine learning, and more. These libraries save a lot of time and even make ugly tasks pretty easy. Libraries like Pandas for easy data manipulation, Django and Flask for web development, and many more.
- Example :
import pandas as pd
data = {"Name": ["Alice", "Bob", "Charlie"], "Age": [24, 30, 35]}
df = pd.DataFrame(data)
print(df)
# Output:
# Name Age
# 0 Alice 24
# 1 Bob 30
# 2 Charlie 35
Python7. Cross-Platform Compatibility – Python is cross-platform, so the same code runs on all three operating systems: Windows, macOS, and Linux. That makes it extremely useful for any portable application building as-long-as you have Python loaded on each of these kinds of platforms, you can run the same Python script across all of them.
- Example :
# A simple "Hello World" program will run on any platform with Python installed.
print("Hello, World!")
Python8. Automatic Memory Management (Garbage Collection) – So, Python has an auto-garbage collector that handles memory allocation and deallocation. Developers therefore do not have to free up memory manually. When an object is no longer in use, memory is freed up from it, thus eliminating memory leaks.
- Example :
class Car:
def __init__(self, model):
self.model = model
car1 = Car("Toyota")
car2 = car1
del car1 # car1 is deleted, but car2 still points to the same Car object
print(car2.model) # Output: Toyota
Python9. Support for Multi-threading and Multiprocessing – It natively supports both multi-threading and multiprocessing. Developers can write programs that execute several tasks at the same time. While the Global Interpreter Lock in the CPython implementation means that CPU-bound threads are not executed in parallel, the module multiprocessing allows for a parallel run across multiple CPU cores, which is of great significance for CPU-intensive tasks. With threading and multiprocessing, Python is adequate of handling I/O-bound and CPU-bound tasks effectively, making it suitable for applications like parallel computations, web servers and data processing pipelines.
- Example using the
Threading
Module :
import threading
import time
def print_numbers():
for i in range(5):
print(i)
time.sleep(1)
def print_letters():
for letter in 'ABCDE':
print(letter)
time.sleep(1)
# Creating threads
t1 = threading.Thread(target=print_numbers)
t2 = threading.Thread(target=print_letters)
# Starting threads
t1.start()
t2.start()
# Waiting for threads to complete
t1.join()
t2.join()
# Output (interleaved):
# 0
# A
# 1
# B
# ...
Python10. Large and Active Community – There are a number of actively contributing developers in Python, with lots of people contributing to its growth and development. This means a lot of resources, libraries, and frameworks are available that can further support in handling your projects.
Limitations of Python
1. Speed – Python being an interpreted language, executes code slower than compiled languages like C or Java.
- Example :
- This will execute slower if compared to C or C++, especially for CPU-intensive tasks.
import time
# Using Python for a large computation
start_time = time.time()
result = sum(i * i for i in range(10**7)) # Computationally heavy
print(f"Execution Time: {time.time() - start_time} seconds")
Python2. Weak in Mobile Development – Python doesn’t have exclusive support for mobile app development when compared to platforms like Kotlin for Android or Swift for iOS.
- Example – Python frameworks like Kivy exist for mobile app development but they are not widely used or optimized for mobile development.
3. Global Interpreter Lock (GIL) – Python’s GIL restricts multithreaded applications by enabling only one thread to run at a particular point of time in a single process, thus performance in multi-core systems is reduced. Multiple threads cannot take full advantage of multi-core processors due to GIL.
- Example :
import threading
def worker():
for _ in range(1000000):
pass
threads = [threading.Thread(target=worker) for _ in range(4)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
Python4. Memory Consumption – Due to Garbage Collection & Dynamic Typing, Python consumes lot of Memory compared to other Languages.
- Example :
- Python objects use more memory than equivalent C structures.
a = [1] * 10**7 # Large list in memory
b = (1,) * 10**7 # Tuple uses less memory
Python5. Dependency on Different Libraries – Most of the Python applications count on external libraries, making them less self-contained.
- Example – Python needs libraries like
matplotlib
orseaborn for Data Visualization
, whereas languages like R have built-in visualization capabilities.
Python in the Modern World: Applications Across Industries
The flexibility of Python has made it an indispensable utility in many fields, allowing a wide range of applications from web development to scientific research. Below points discuss the use of Python in several industries:
1. Web Development – Python has very powerful frameworks like Flask, Django and FastAPI that simplify web application development. These frameworks provide important tools for handling authentication, routing, database integration and much more.
- Real-World Applications: Companies like Instagram, Spotify, and Pinterest use Python for back-end development. For example, Instagram’s backend runs on Django; this allows it to process millions of user interactions and media content efficiently.
- Example :
from flask import Flask
app = Flask(__name__)
@app.route("/")
def home():
return "Welcome to the homepage!"
if __name__ == "__main__":
app.run()
Python2. Data Science and Data Analysis – Python’s rich ecosystem includes libraries like Pandas (data manipulation), NumPy (numerical computing), and Matplotlib (data visualization), making it favorite choice of data scientists.
- Real-World Applications: Industries in marketing, finance, healthcare, and retail use Python for analyzing customer data, trend analysis and financial forecasting. Netflix, for example, uses Python for data analysis to personalize content recommendations and optimize content delivery.
- Example :
import pandas as pd
data = {"Sales": [300, 400, 500, 600], "Profit": [50, 80, 100, 120]}
df = pd.DataFrame(data)
print(df.describe()) # Summary statistics for sales and profit data
Python3. Machine Learning and Artificial Intelligence – Python provides famous machine learning and AI libraries such as Keras, PyTorch, TensorFlow and scikit-learn. These libraries support everything from simple machine learning algorithms to deep learning models.
- Real-World Applications: Python is used in industries like finance (for fraud detection), e-commerce (for personalized recommendations) and healthcare (for diagnostics and medical imaging). For instance, Netflix and Amazon use machine learning models built with Python to personalize user recommendations.
- Example :
from sklearn.linear_model import LinearRegression
import numpy as np
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1, 3, 2, 3, 5])
model = LinearRegression()
model.fit(X, y)
print(model.predict([[6]])) # Predict the value for input 6
Python4. Automation and Scripting – Python is often used to automate repetitive tasks such as data entry, web scraping and file management. Libraries like Selenium, BeautifulSoup and Requests make it easy to interact with web pages and automate browser actions.
- Real-World Applications: Python scripts are used in IT departments for tasks like automating backups, managing servers and monitoring network security. Applications like Reddit use Python for content moderation and web scraping.
- Example :
from bs4 import BeautifulSoup
import requests
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title.text) # Extract and print the title of the webpage
Python5. Cybersecurity – Python is used to develop cybersecurity tools, such as, malware analysis, vulnerability scanning and penetration testing. Libraries like Requests and Scapy assist in HTTP requests and network scanning, respectively.
- Real-World Applications: Cybersecurity professionals and firms use Python for scripting security tools and developing intrusion detection systems. Python scripts automate tasks like network scanning, log analysis and exploit development.
- Example :
import socket
# Simple port scanner
def scan_port(ip, port):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.settimeout(1)
result = sock.connect_ex((ip, port))
sock.close()
return result == 0
print(scan_port("127.0.0.1", 80)) # Check if port 80 is open
Python6. Healthcare and Bioinformatics – Python libraries like Pandas and Biopython are used to process and analyze biological data, such as protein structures and DNA sequences.
- Real-World Applications: Python is applied in bioinformatics for medical image analysis, genome analysis and drug discovery. For example, Python is used in genomics research for DNA sequencing and data visualization in medical studies.
- Example :
from Bio.Seq import Seq
dna_seq = Seq("AGTACACTGGT")
print(dna_seq.complement()) # Output: TCATGTGACCA (complementary DNA strand)
Python7. Finance and Fintech – Python is widely used for risk management, quantitative and algorithmic trading and predictive analysis. Libraries like Pandas and NumPy help with financial data analysis, while TA-Lib and QuantLib are used for financial modeling.
- Real-World Applications: Python is used in banking systems, trading platforms and investment firms to analyze financial trends, conduct backtesting, and automate trading strategies. Goldman Sachs and J.P. Morgan are examples of financial institutions who use Python extensively.
- Example :
import pandas as pd
# Analyze stock price trends
stock_data = {"Day": [1, 2, 3, 4], "Price": [100, 102, 104, 103]}
df = pd.DataFrame(stock_data)
print(df.diff()) # Calculate daily price changes
Python8. Scientific Computing and Research – Python is heavily used in research due to libraries like NumPy, SciPy and Matplotlib. For data visualization, Seaborn and Matplotlib are essential, while SymPy allows symbolic mathematics.
- Real-World Applications: Python is used by organizations like NASA for scientific computing, data analysis and simulation in fields such as physics, engineering and astronomy.
- Example :
import numpy as np
# Scientific calculation
matrix = np.array([[1, 2], [3, 4]])
print(np.linalg.inv(matrix)) # Calculate the inverse of the matrix
PythonReference Videos :
These NOTES dwells on defining Python, its historical evolution since it was first created by Guido van Rossum in 1991 to date, and its general-purpose and widespread use as a programming language. We considered the top features of Python, such as simplicity, readability, cross-platform compatibility, a huge standard library, and interfacing with other technologies supported with appropriate examples including some of its Limitations . We also looked at some real-world applications of Python across various industries: web development, data science, machine learning, automation, finance, etc. Python adaptation, good community support, and a non-steep learning curve make it perfectly suitable for beginners and professionals dealing with complex challenges in software development.