In Java, Keywords are reserved words that hold special meaning for the Java compiler. These words are predefined in the Java programming language and serve a specific function. Keywords are integral to the syntax of Java, and each keyword has a defined purpose, such as controlling program flow, defining data types, or specifying access levels for classes and methods. Since these words are reserved by the language, you cannot use them as identifiers (e.g., variable names or class names).
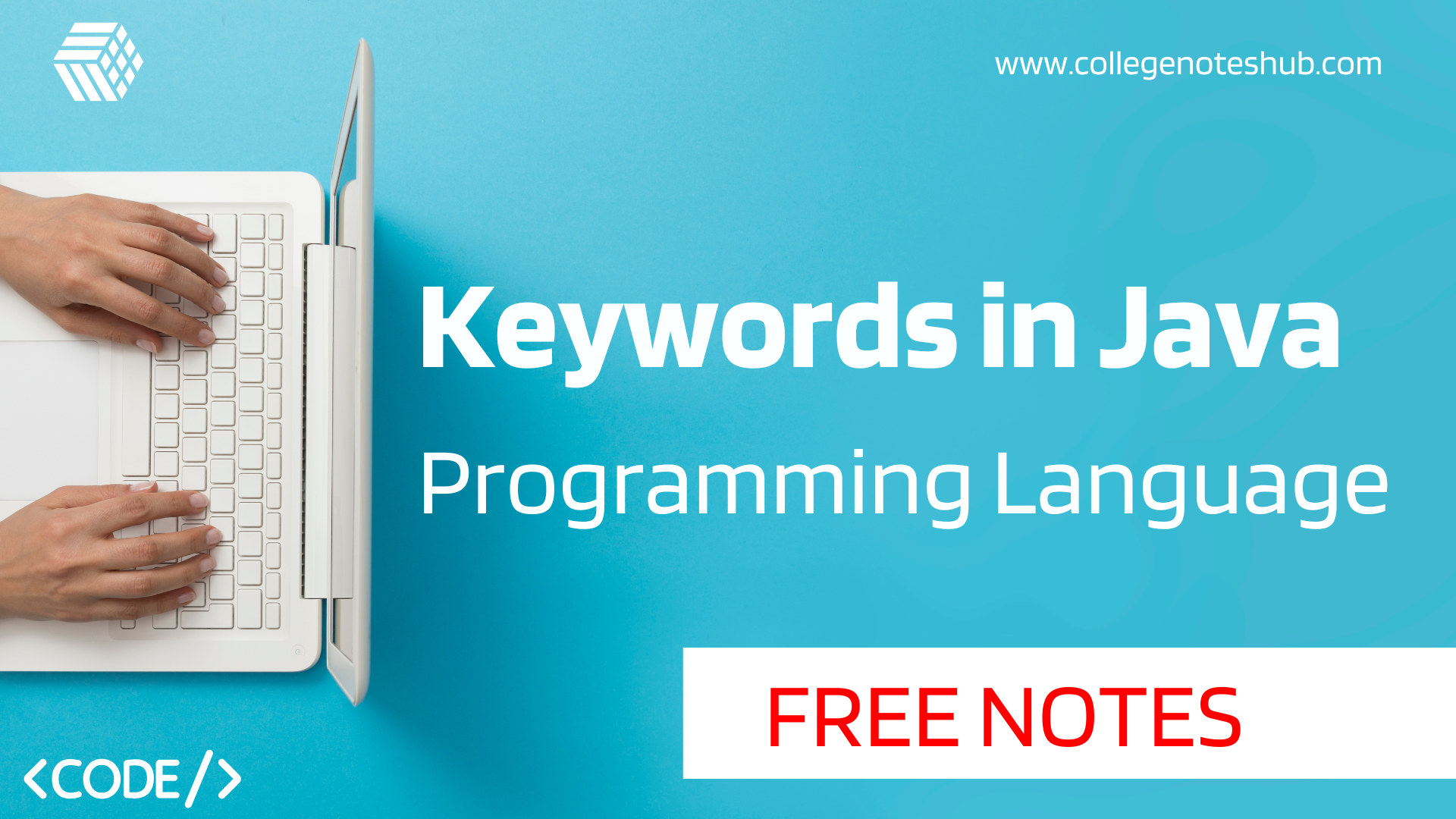
Table of Contents
What Are Java Keywords?
Java keywords are a set of predefined words that the Java compiler recognizes as having a special meaning. They are fundamental to the Java programming language and are part of its grammar and structure. Keywords can’t be used for anything other than their intended purposes. In total, Java contains 50 reserved keywords, and each of them performs a specific function within a program.
Keywords in Java are case-sensitive, meaning that they must always be written in lowercase. For example, the keyword public
is valid, but Public
or PUBLIC
would not be recognized as a keyword by the compiler.
Role of Java Keywords
Java keywords allow developers to define:
- Control flow structures like loops and conditionals.
- Access modifiers to control the visibility of variables and methods.
- Data types for variables and constants.
- Exception handling to deal with errors in a controlled way.
- Class and object manipulation, including inheritance and polymorphism.
Without these keywords, Java would not have the structure and functionality required to manage objects, control program flow, and handle data types.
Categories of Java Keywords
Java keywords can be classified into several categories based on their use. These categories help organize the keywords and make their roles clearer to developers. Some of the primary categories include:
- Control Flow Keywords
- Access Modifiers
- Data Type Keywords
- Class and Object Related Keywords
- Exception Handling Keywords
- Other Miscellaneous Keywords
Let’s dive deeper into these categories:
1. Control Flow Keywords
Control flow keywords in Java dictate the order in which statements in the program are executed. They are essential for making decisions, repeating actions, and controlling the program’s flow based on certain conditions. Some examples of control flow keywords are:
if
,else
: These keywords allow for conditional execution based on a test condition.switch
,case
: These provide a multi-way branch, making it easier to handle multiple conditions in a compact way.break
: Used to exit a loop or aswitch
statement prematurely.continue
: Skips the current iteration of a loop and moves on to the next one.return
: Exits a method and returns a value, if applicable.
Example:
int number = 5;
if (number > 0) {
System.out.println("Positive number");
} else {
System.out.println("Negative number");
}
Java2. Access Modifier Keywords
Java access modifiers control the visibility of classes, methods, and variables. By specifying the access level, you can limit access to certain components of your program, enhancing security and modularity.
public
: Specifies that the class, method, or variable is accessible from anywhere in the program.private
: Restricts access to the class, method, or variable to within its own class.protected
: Allows access within the same package and by subclasses.default
: When no access modifier is specified, the default access level is package-private, meaning it is accessible only within the same package.
Example:
public class Example {
private int number; // private variable
public void setNumber(int num) { // public method
number = num;
}
}
Java3. Data Type Keywords
Java’s data types can be divided into primitive and non-primitive types. Java keywords for data types allow you to declare variables and constants of various types. These data types define the kind of data a variable can hold.
int
,char
,boolean
,float
,double
,long
,byte
,short
: These are the primitive data types.void
: Specifies that a method does not return a value.
Example:
int age = 30; // Primitive data type: int
boolean isActive = true; // Primitive data type: boolean
void greet() { // void method (does not return any value)
System.out.println("Hello");
}
Java4. Class and Object Related Keywords
Java is an object-oriented language, and many keywords relate to defining classes, objects, and inheritance. These keywords allow you to work with the core principles of object-oriented programming, such as encapsulation, inheritance, and polymorphism.
class
: Used to declare a new class.interface
: Used to declare an interface.extends
: Specifies that a class inherits from a superclass.implements
: Used when a class implements an interface.new
: Used to create new objects.
Example:
public class Animal {
void makeSound() {
System.out.println("Animal sound");
}
}
public class Dog extends Animal { // Dog inherits from Animal class
void makeSound() {
System.out.println("Bark");
}
}
Java5. Exception Handling Keywords
Java provides powerful exception-handling mechanisms to deal with runtime errors. These keywords allow developers to handle exceptions, ensuring that the program can continue running even when an error occurs.
try
: Defines a block of code to test for exceptions.catch
: Defines a block to handle exceptions that arise in thetry
block.finally
: Always executes aftertry
andcatch
blocks, regardless of whether an exception occurred.throw
: Used to explicitly throw an exception.throws
: Declares exceptions that a method might throw.
Example:
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Error: Cannot divide by zero");
} finally {
System.out.println("This will always execute");
}
Java6. Other Miscellaneous Keywords
Java includes several keywords that don’t fit neatly into other categories, but are still important for certain functionalities:
this
: Refers to the current instance of the class.super
: Refers to the superclass of the current object.static
: Denotes that a method or variable belongs to the class rather than to an instance of the class.final
: Used to define constants, prevent method overriding, and prevent inheritance.synchronized
: Ensures that a method or block is accessed by only one thread at a time.volatile
: Indicates that a variable’s value can be changed unexpectedly by multiple threads.
Example:
public class Parent {
public void showMessage() {
System.out.println("Message from Parent");
}
}
public class Child extends Parent {
@Override
public void showMessage() {
super.showMessage(); // Calls method from Parent
System.out.println("Message from Child");
}
}
JavaList of Java Keywords
Here is a list of all 50 Java keywords:
Keyword | Keyword | Keyword | Keyword |
---|---|---|---|
abstract | assert | boolean | break |
byte | case | catch | char |
class | const | continue | default |
do | double | else | enum |
extends | final | finally | float |
for | goto | if | implements |
import | instanceof | int | interface |
long | native | new | null |
package | private | protected | public |
return | short | static | strictfp |
super | switch | synchronized | this |
throw | throws | transient | try |
void | volatile | while |
Youtube Links :
Java keywords form the foundation of the language’s syntax. By understanding and correctly using these reserved words, you can efficiently write Java programs that follow the language’s structure and conventions. Whether dealing with data types, class structures, control flow, or exception handling, keywords define the way Java programs are written and executed. As a Java developer, mastering these keywords is essential for building robust and maintainable applications.