Java is a popular programming language. Developers use it for many purposes, like creating mobile apps and large software programs. Its main strength is its ability to work on different devices. This “write once, run anywhere” feature lets Java code run on any device that has a Java Virtual Machine (JVM). Because of this, Java is both flexible and dependable.
In this article, we’ll explore Java’s background, key features, a “Hello World” example, comparisons with C++ and Python, and the difference between byte code and machine code.
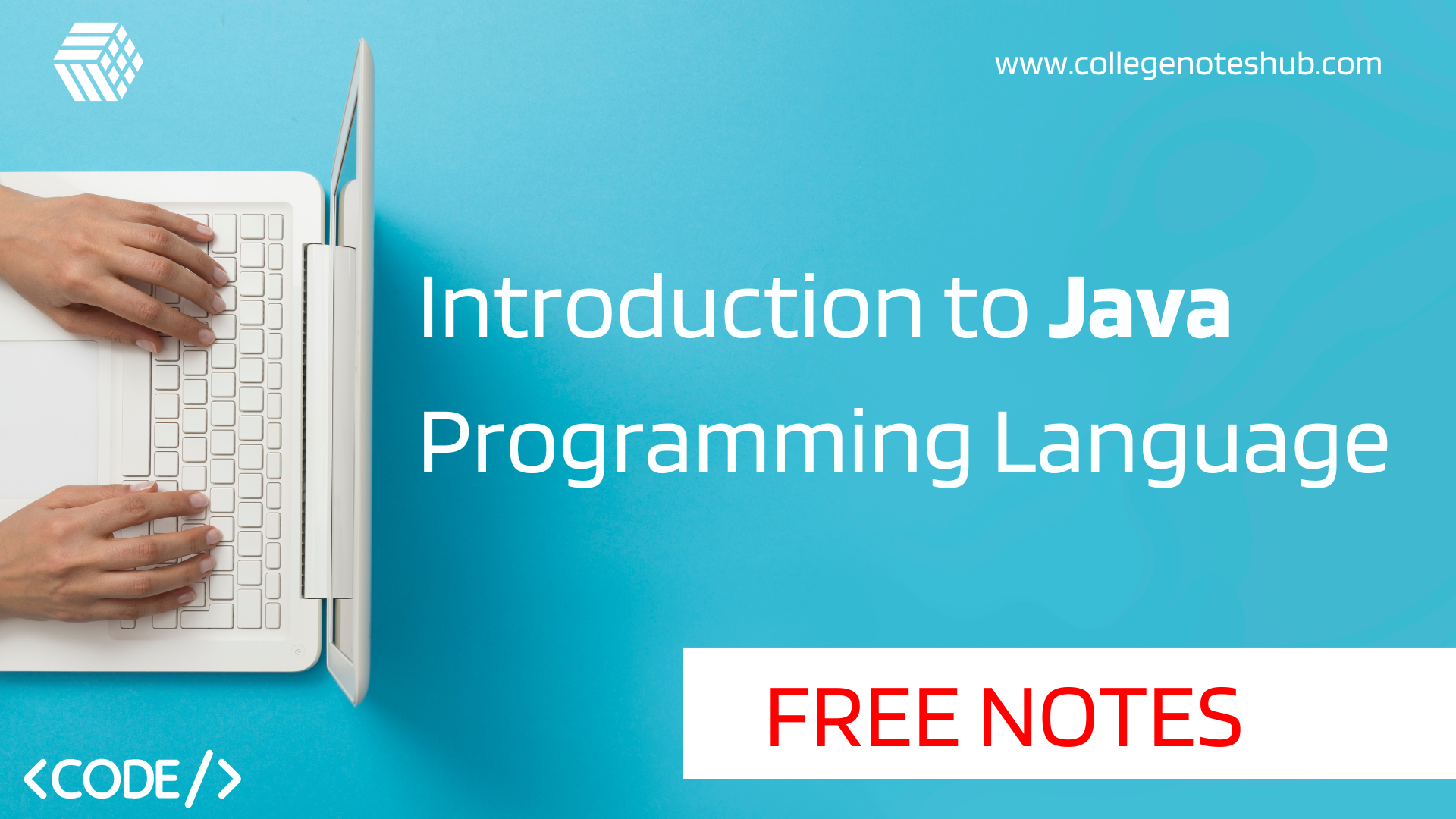
1. History of Java
Java started in the early 1990s. Sun Microsystems wanted a programming language that could run on different types of devices without needing any changes. This goal led to the creation of Java.
Java’s Timeline:
- 1991: James Gosling, Mike Sheridan, and Patrick Naughton led a project called the “Green Project.” Their goal was to create a language that would work on many devices, like TVs and home appliances.
- 1995: Java was officially released as Java 1.0. Its special virtual machine made it possible to run the same code on different devices. This idea became known as “write once, run anywhere.”
- 2009: Oracle acquired Sun Microsystems, and Java became part of Oracle’s software family. Since then, Oracle has kept Java up-to-date, focusing on security, speed, and reliability.
- Today: Java is widely used in many industries, from finance to mobile development. Because Java is so reliable, it’s often chosen for large applications.
2. Features of Java
Java offers many features that make it powerful and useful for developers. Here are its most important qualities:
- Platform Independence: Java works across many platforms. It compiles code into byte code, which runs on any device with a JVM. This makes Java versatile.
- Object-Oriented: Java follows an object-oriented programming style (OOP). This approach lets developers organize and reuse code. It is especially helpful for complex projects.
- Robust and Secure: Java has strong tools for handling errors and protecting code. It prevents many common problems in programming, making it a safe choice for applications that need security.
- Multithreading Support: Java can handle several tasks at once, thanks to multithreading. This feature is especially helpful for programs that must manage many things at the same time, like web servers.
- Automatic Memory Management: Java includes a garbage collector. This system automatically frees up memory by removing data that is no longer needed. This feature makes Java applications run smoothly.
- High Performance: Java uses a Just-In-Time (JIT) compiler, which speeds up execution by converting byte code into machine code while the program runs. This process makes Java faster than many interpreted languages.
3. Hello World Program with Java
A “Hello World” program is the simplest way to learn a new language. Here’s an example in Java:
public class HelloWorld
{
public static void main(String[] args)
{
System.out.println("Hello, World!");
}
}
JavaExplanation:
public class HelloWorld
: This line defines a class namedHelloWorld
. In Java, every program begins with a class.public static void main(String[] args)
: This is the main method. The JVM starts running the code here.System.out.println("Hello, World!");
: This line displays “Hello, World!” on the screen. It’s a small but useful first step for learning Java.
4. Java vs C++ vs Python
Java, C++, and Python are all popular programming languages. However, each language has unique strengths. Here’s a quick look at their main differences:
Feature | Java | C++ | Python |
---|---|---|---|
Syntax | Clear, though somewhat long | Complex, especially with pointers | Simple and easy to read |
Performance | Fast, but slower than C++ | Very fast, ideal for high-performance needs | Slower due to interpretation |
Memory Management | Automatic (garbage collection) | Manual, allowing more control | Automatic (garbage collection) |
Use Cases | Apps, Android development | System software, games, real-time tasks | Data science, automation, web dev |
Object-Oriented | Fully object-oriented | Multi-paradigm | Multi-paradigm |
Portability | Highly portable with JVM | Platform-dependent | Very portable with virtual environments |
Examples in Practice: Java works well for large applications that need to run on many platforms. C++ is often better when performance is a priority, like in games and real-time systems. Python, with its simple syntax, is widely used in data science, machine learning, and automation.
Each language has its own version of a “Hello World!” program. Java’s structure may look a bit more complex than Python’s, but it works well for bigger projects.
5. Byte Code vs Machine Code
Java uses a unique method to execute code. Unlike some other languages, it doesn’t compile directly to machine code. Instead, Java uses byte code.
- Byte Code:
- The Java compiler translates Java code into byte code, which it saves in
.class
files. Byte code can run on any device with a JVM, which makes Java platform-independent. - The JVM either interprets or JIT-compiles byte code into machine code during runtime. This flexibility allows Java to work on many different devices.
- The Java compiler translates Java code into byte code, which it saves in
- Machine Code:
- Machine code is low-level code that a computer’s processor understands directly.
- For example, C++ compiles directly into machine code, making it faster but less adaptable. C++ programs often need to be recompiled for each platform.
When Java code is compiled, it first becomes byte code. Then, the JVM interprets or JIT-compiles this byte code to machine code while running. In contrast, C++ compiles straight-to-machine code, making it faster but limited to specific platforms.
Youtube Links :
Java is a widely-used, high-level programming language known for its simplicity, portability, and versatility. It follows the “write once, run anywhere” philosophy, meaning that Java code, once written, can run on any device with a Java Virtual Machine (JVM), making it platform-independent. Java is object-oriented, which helps developers organize and reuse code effectively. It is also known for its robustness, security features, and automatic memory management through garbage collection. With its extensive libraries and frameworks, Java is used in a variety of applications, from mobile development (especially Android apps) to large-scale enterprise systems, and continues to be one of the most popular languages in the software development industry.