Java is a powerful and flexible programming language used widely by developers. Starting with Java requires an understanding of its fundamental components, including syntax, comments, identifiers, keywords, class structure, input/output handling, and writing your first program. Let’s explore each of these basics to build a solid foundation in Java programming.
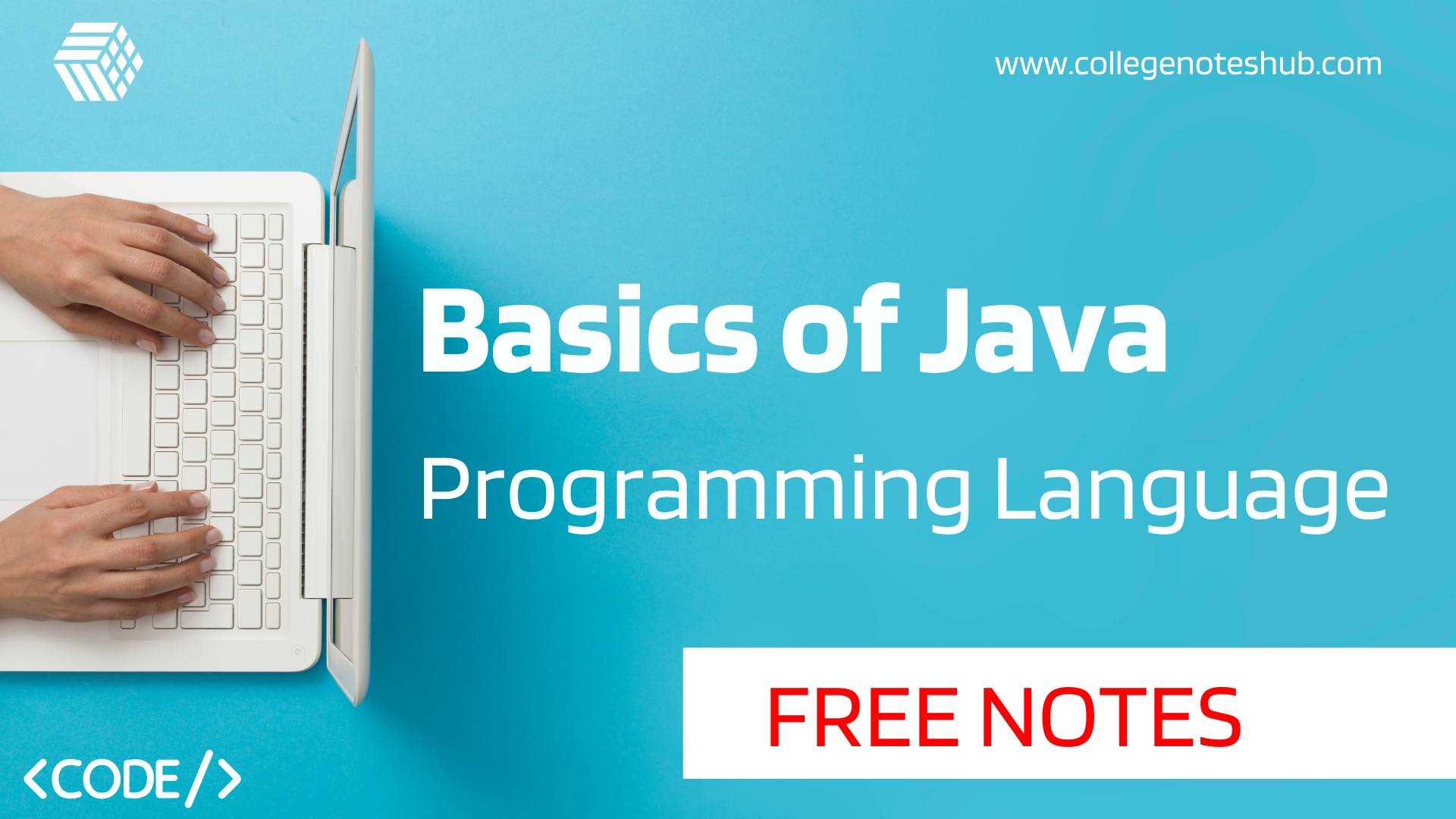
Table of Contents
1. Java Basic Syntax
Java’s syntax is a set of rules that define how code should be written and organized. Each statement in Java ends with a semicolon (;
), and Java is case-sensitive, so Example
and example
would be treated as different identifiers. Curly braces {
}
are used to group blocks of code, like methods and loops. These rules are essential for all Java code.
Example:
public class MyProgram
{
public static void main(String[] args)
{
System.out.println("Hello, Java!");
}
}
JavaIn this example:
public class MyProgram
: Defines a class namedMyProgram
.public static void main(String[] args)
: The main method, where the program starts.System.out.println("Hello, Java!");
: Prints “Hello, Java!” to the console.
2. Comments
Comments make Java code easier to understand. They’re ignored by the compiler and don’t affect the program’s execution. Java supports three types of comments:
Single-Line Comments: Start with //
and go to the end of the line. These are useful for short explanations of the code.
// Print a message to the console
System.out.println("Hello, world!");
JavaMulti-Line Comments: Start with /*
and end with */
. They’re helpful for longer explanations or temporarily disabling code sections.
/* This is a multi-line comment that spans multiple lines */ System.out.println("Welcome to Java!");
JavaDocumentation Comments: Start with /**
and end with */
. These are used for generating structured documentation with Javadoc
/** * This method prints a greeting. */
public void greet() { System.out.println("Hello!"); }
Java– Using comments keeps your code organized and easy to read.
3. Identifiers
Identifiers are names used for variables, methods, classes, and other program elements. They must follow specific rules:
- Begin with a letter (A-Z or a-z), an underscore (
_
), or a dollar sign ($
). - After the first character, they may include numbers.
- Keywords like
int
orclass
cannot be used as identifiers.
Good identifiers make code clear and readable.
Example:
int age = 25; // 'age' is an identifier for a variable
String name = "Alice"; // 'name' is an identifier for a variable
Java4. Keywords
Java uses keywords that have special meanings and functions within the language. They cannot be used as identifiers. Each keyword allows Java to perform different operations and define the program structure.
Some common keywords:
public
: Controls visibility.class
: Defines a class.int
: Declares an integer variable.if
/else
: Used for conditional statements.return
: Ends a method and returns a value if specified.
Example:
public class Example {
public static void main(String[] args)
{
int number = 10;
if (number > 5)
{
System.out.println("Number is greater than 5");
}
}
}
JavaIn this example:
int
declares an integer variable.if
is used for a conditional statement.
5. Class Syntax
In Java, everything exists within classes. A class serves as a blueprint for objects and contains variables, methods, and constructors. Each Java program must contain at least one class, which includes the entry point of the program, the main
method.
Example of Class Syntax:
public class MyClass {
int myVariable; // Class variable
public MyClass() { // Constructor
myVariable = 0;
}
public void display() { // Method
System.out.println("Hello from Java!");
}
public static void main(String[] args) { // Entry point of the program
MyClass obj = new MyClass();
obj.display();
}
}
JavaIn this example:
public class MyClass
: Declares a class namedMyClass
.int myVariable;
: A variable declared within the class.display()
: A method that prints a message.public static void main(String[] args)
: The main method, where Java starts running the program.
Classes are the foundation of Java programs, making them essential to learn.
6. Input/Output in Java
Java handles input and output (I/O) operations to make programs interactive. The System.out.println()
method displays messages on the console, and the Scanner
class reads user input.
Output Example:
System.out.println("Enter your name:");
JavaInput Example:
import java.util.Scanner;
public class UserInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in); // Create a Scanner object
System.out.print("Enter your age: ");
int age = scanner.nextInt(); // Reads an integer from user input
System.out.println("You are " + age + " years old.");
}
}
JavaIn this example:
System.out.println("Enter your name:");
: Prints a message to the console.Scanner scanner = new Scanner(System.in);
: Creates aScanner
object to read input.scanner.nextInt();
: Reads an integer from user input.
Input and output are key for creating interactive applications.
7. First Java Program (Hello World)
The “Hello World” program is often the first program in Java. This simple program shows Java’s structure and syntax and outputs “Hello, World!” to the console. It’s a good starting point for understanding Java’s setup.
Hello World Example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
JavaExplanation:
public class HelloWorld
: Declares a class namedHelloWorld
.public static void main(String[] args)
: The main method where Java begins program execution.System.out.println("Hello, World!");
: Prints “Hello, World!” to the console.
This first program introduces Java’s basic structure and syntax, making it an essential starting point.
Youtube Videos:
The basics of Java include understanding its syntax, comments, identifiers, keywords, and class structure. Java’s syntax provides the rules for writing code, while comments improve readability. Identifiers are names for variables, methods, and classes, and keywords define specific functions. Java programs revolve around classes, each containing methods like main
for execution. These essentials form the foundation of Java programming, enabling developers to write efficient, organized code.