In this article, we’ll take you through the complete structure of a C program, from the foundational components like preprocessor directives, the main function, and variable declarations, to essential concepts like statements, expressions, and control structures. You will also learn about loops, function calls, and how the C compilation & execution process works. With practical code examples and clear explanations, this guide is perfect for anyone looking to master the building blocks of C programming. Get ready to dive in and start writing efficient, well-structured C code!
Structure of a C Program
The structure of a C program is divided into several essential components. These include the syntax, control structures, and core elements that form the backbone of C programming. Below is an overview of the basic structure of a C program:
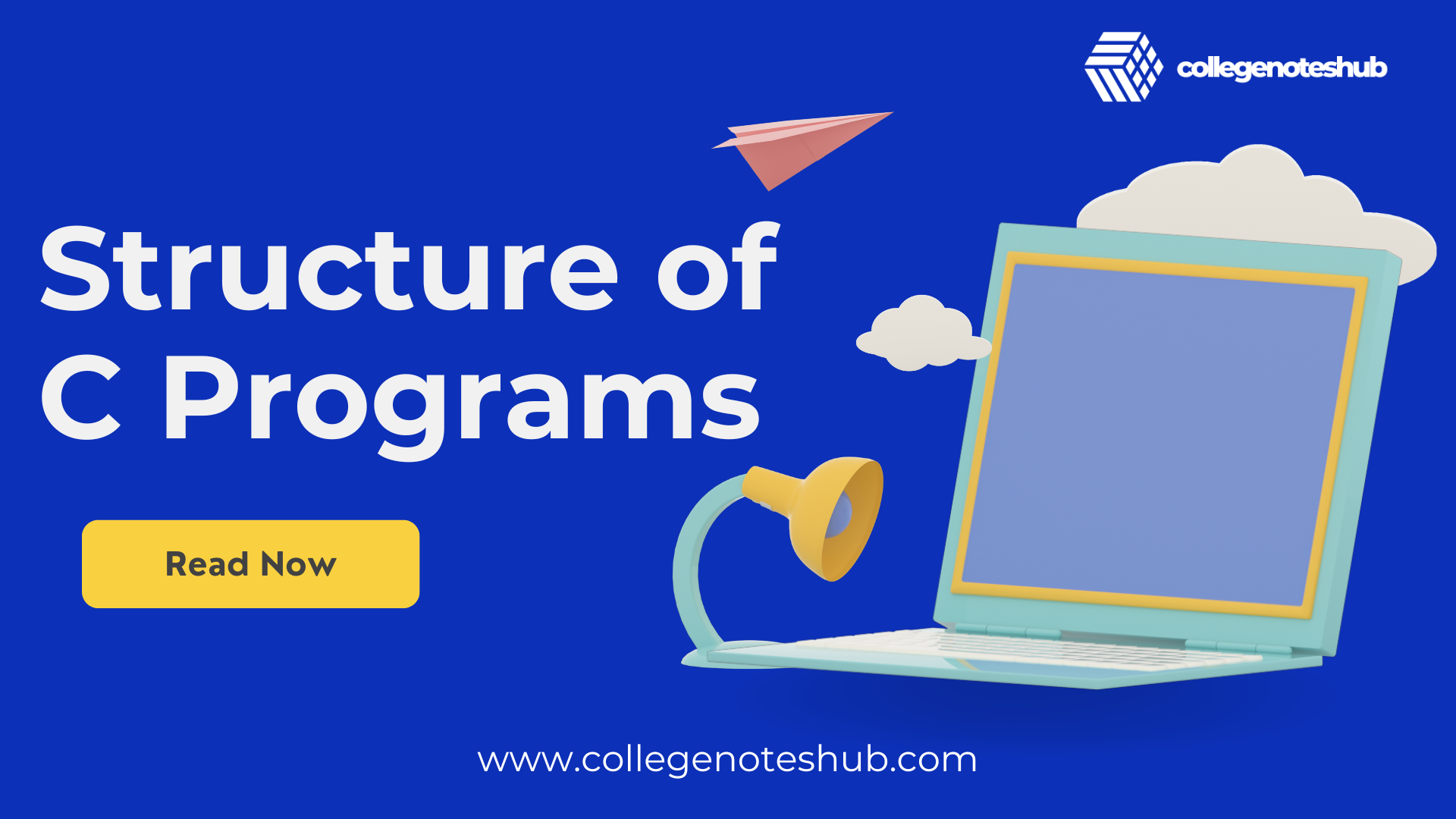
- Preprocessor Directives
- Main Function
- Variable Declaration
- Statements and Expressions
- Control Structures
- Functions
1. Preprocessor Directives
Preprocessor directives are instructions given to the C compiler before the actual compilation process begins. They control the compilation process, include external files, define constants, and handle macros.
#include: This directive includes the contents of another file into the current file.
#include <stdio.h> // Includes the standard I/O library
#include "myheader.h" // Includes a user-defined header file
C#define: Defines macros or constants, allowing the assignment of names to constant values or code snippets.
#define PI 3.14159 // Defines a constant PI
#define MAX(a, b) ((a) > (b) ? (a) : (b)) // Macro to get the maximum of two numbers
C#undef: Undefines a macro.
#define TEMP 100 #undef TEMP // TEMP is now undefined and can be redefined
C#ifdef / #ifndef: Conditional compilation based on whether a macro is defined or not.
#define DEBUG 1
#ifdef DEBUG printf("Debug mode is on\n");
#endif
#ifndef RELEASE
printf("This is not a release version\n");
#endif
C#if, #else, #elif, #endif: Conditional compilation based on a constant expression
#define VERSION 2
#if VERSION == 1 printf("Version 1\n");
#elif VERSION == 2 printf("Version 2\n");
#else printf("Unknown version\n");
#endif
C#error: Forces a compilation error with a custom message. #error "Invalid input"
#pragma: Compiler-specific directives to control warnings and behavior. #pragma once // Ensures a header file is included only once
2. Main Function
The main()
function is the entry point of any C program. It controls the program flow and typically calls other functions.
int main() {
// Code logic goes here
return 0;
}
C3. Variable Declaration in C
Variables store data values and must be declared before use. Each variable declaration specifies the data type and optionally initializes a value.
int x = 10; // Declares an integer variable
char letter = 'A'; // Declares a character variable
CCommon data types include:
- int: Integer values
- float: Floating-point numbers
- double: Double-precision floating-point numbers
- char: Single characters
4. Statements and Expressions
Statements in C represent actions that the program executes. These include:
- Declaration Statements: Used to declare variables, functions, etc.
int a, b;
- Assignment Statements: Assign values to variables.
a = 10;
- Control Flow Statements: Control the order of execution.
if (a > 0) { printf("Positive number"); }
- Function Call Statements: Call functions to perform specific tasks.
printf("Hello, World!\n");
Expressions are combinations of variables, constants, and operators that evaluate to a value.
int result = 2 * 3 + 5; // Arithmetic expression
int max = (x > y) ? x : y; // Conditional expression
C5. Control Structures
Control structures in C guide the program’s flow and decision-making.
Sequential: Statements execute in the order they are written.
if (condition)
{
// Code to execute if condition is true
}
else
{
// Code to execute if condition is false
}
CConditional: Executes code based on a condition.
Switch: Selects code to execute based on a variable’s value.
switch (expression)
{
case value1:
// Code for value1
break;
case value2:
// Code for value2
break;
default: // Default case
}
CLoops: Repeat a block of code multiple times.
For loop: Useful when the number of iterations is known.
for (int i = 0; i < 5; i++) { printf("%d\n", i); }
CWhile loop: Repeats while a condition is true.
while (condition) { // Code to execute while condition is true }
CDo-While loop: Executes the code block at least once, then repeats while a condition is true.
do
{
// Code to execute
}
while (condition);
CJump Statements: Direct the flow abruptly.
break: Exits a loop or switch statement.
for (int i = 0; i < 10; i++)
{
if (i == 5) break;
}
Ccontinue: Skips the rest of the loop’s iteration.
for (int i = 0; i < 10; i++)
{
if (i == 5) continue;
}
Cgoto: Jumps to a labeled statement (generally discouraged).
if (condition)
goto label;
label:
// Code to jump to
C6. Functions
Functions are reusable blocks of code that perform a specific task. Every C program contains at least one function, main()
, but more can be defined.
int add(int a, int b) {
return a + b;
}
int main() {
int sum = add(5, 3);
printf("Sum: %d", sum);
return 0;
}
CCompilation and Execution Process in C
The C compilation and execution process converts source code into an executable program through multiple stages:
- Preprocessing: Handles directives like
#include
and#define
. - Compilation: Translates preprocessed code into assembly code.
- Assembly: Converts assembly code into machine code, generating object files.
- Linking: Combines object files and libraries to create the final executable.
- Execution: The operating system loads the executable into memory and runs it, with the output shown on the user’s screen.
Reference Links to Youtube Lectures :
You’ve just uncovered the essential structure of a C program, including how to use preprocessor directives, manage variables, and control program flow with loops and conditions. This article has laid a solid foundation for your C programming journey, making complex concepts more approachable with real-world examples. Be sure to check out our next article, where we’ll dive even deeper into advanced C programming techniques. Keep reading and sharpening your skills as you continue to explore the incredible world of C programming!