The Structure of C program is fundamental to understand how to write code effectively. This section will outline the basic syntax of a C program, including its structure, syntax, and examples :
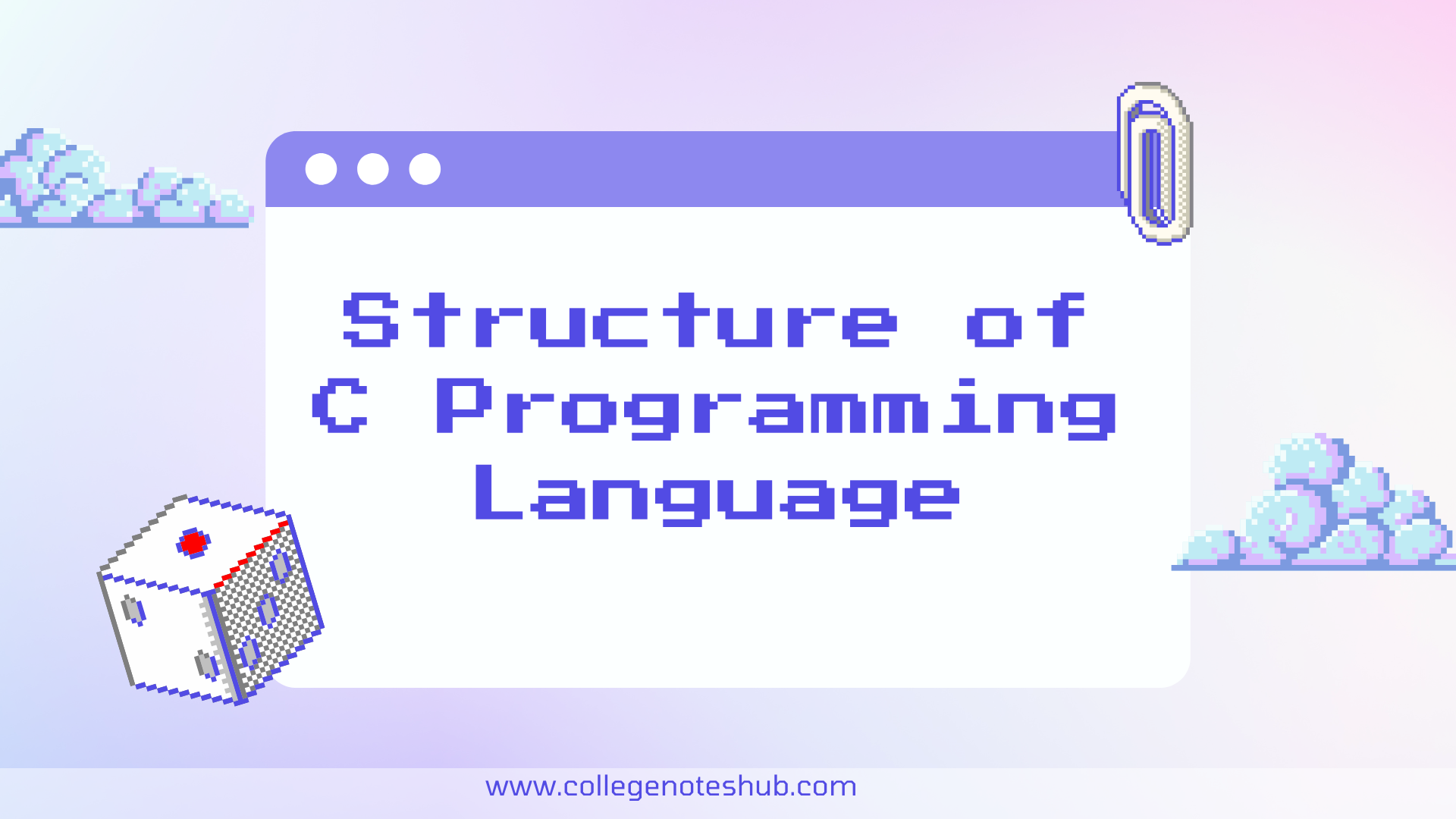
Table of Contents
What is the Structure of C ?
The Structure of a C program refers to the arrangement of various elements that make up the program, including preprocessor directives, function declarations, variable declarations, and statements. Each part plays a specific role in the program’s functionality and overall organization.
Syntax of C
The syntax of C programming refers to the set of rules that defines the combinations of symbols and keywords that are used in a C Program. Understanding the syntax is crucial for writing valid and functional C codes.
Example of Syntax :
#include <stdio.h> // Preprocessor directive
// Main function - execution starts here
int main() {
// Variable declarations
data_type variable_name;
// Statements
statement1;
statement2;
// ...
return value; // Return statement
}
CKey Elements of C Syntax
- Preprocessor Directives:
- Syntax:
#include <header_file.h>
- Used to include standard libraries or other header files before the compilation of the program.
- Syntax:
- Function Declarations:
- Syntax:
return_type function_name(parameter_list);
- Declares a function, specifying its return type and parameters (if any).
- Syntax:
- Main Function:
- Syntax:
int main() { ... }
- The entry point of a C program where execution begins.
- Syntax:
- Variable Declarations:
- Syntax:
data_type variable_name;
- Declares a variable of a specified data type.
- Syntax:
- Statements:
- Syntax:
statement1; statement2;
- Executable instructions that must end with a semicolon (
;
).
- Syntax:
- Comments:
- Single-line comment:
// This is a single-line comment
- Multi-line comment:
/* This is a multi-line comment */
- Comments are used to document code and are ignored by the compiler.
- Single-line comment:
- Data Types:
- Common data types include:
int
: for integersfloat
: for floating-point numbersdouble
: for double-precision floating-point numberschar
: for characters
- Common data types include:
- Operators:
- Arithmetic operators:
+
,-
,*
,/
,%
- Relational operators:
==
,!=
,>
,<
,>=
,<=
- Logical operators:
&&
,||
,!
- Arithmetic operators:
We’ll discuss about them in detail one by one in our upcoming sections.
Some more Examples of C
Here are some basic C Programs which are taught in College :
1. Adding Two Numbers
This program takes two integers as input and prints their sum.
#include <stdio.h>
int main() {
int num1, num2, sum;
// Input two numbers
printf("Enter first number: ");
scanf("%d", &num1);
printf("Enter second number: ");
scanf("%d", &num2);
// Calculate sum
sum = num1 + num2;
// Display the result
printf("Sum: %d\n", sum);
return 0;
}
C2. Printing Numbers from 1 to 10
This program prints the numbers from 1 to 10 using a loop.
#include <stdio.h>
int main() {
int i;
// Print numbers from 1 to 10
printf("Numbers from 1 to 10:\n");
for (i = 1; i <= 10; i++) {
printf("%d\n", i);
}
return 0;
}
C3. Finding the Largest of Three Numbers
This program takes three numbers as input and determines the largest among them.
#include <stdio.h>
int main() {
int num1, num2, num3;
// Input three numbers
printf("Enter three numbers:\n");
scanf("%d %d %d", &num1, &num2, &num3);
// Determine the largest number
if (num1 >= num2 && num1 >= num3) {
printf("Largest number: %d\n", num1);
} else if (num2 >= num1 && num2 >= num3) {
printf("Largest number: %d\n", num2);
} else {
printf("Largest number: %d\n", num3);
}
return 0;
}
C4. Calculating the Factorial of a Number
This program calculates the factorial of a given number using a loop.
#include <stdio.h>
int main() {
int num, i;
unsigned long long factorial = 1; // Use long long for larger numbers
// Input a number
printf("Enter a positive integer: ");
scanf("%d", &num);
// Calculate factorial
for (i = 1; i <= num; i++) {
factorial *= i;
}
// Display the result
printf("Factorial of %d = %llu\n", num, factorial);
return 0;
}
C5. Reversing a Number
This program reverses a given integer.
#include <stdio.h>
int main() {
int num, reversedNum = 0, remainder;
// Input a number
printf("Enter an integer: ");
scanf("%d", &num);
// Reverse the number
while (num != 0) {
remainder = num % 10; // Get the last digit
reversedNum = reversedNum * 10 + remainder; // Build the reversed number
num /= 10; // Remove the last digit
}
// Display the result
printf("Reversed Number: %d\n", reversedNum);
return 0;
}
CThese basic C programs introduce fundamental programming concepts such as input/output, loops, conditionals, and arithmetic operations. As you practice these programs, you’ll gain a better understanding of how to structure and write C code effectively.
Youtube Reference Links :
Understanding the syntax of C is essential for writing valid programs. By adhering to these rules and conventions, programmers can create effective and efficient code. In upcoming posts, we will explore specific features of C, including control structures, data types, and functions.