C Programming Language is the most basic programming language. It is the foundation for many modern language systems & frameworks. It was developed by Dennis Ritchie at Bell Labs in the early 1970s. C Language is known for its simplicity and efficiency. It’s influence extends to many modern languages like C++, Java, and Python. Learning C helps you understand fundamental programming concepts and gives you control over hardware and system resources. This series will guide you through C programming, from basic syntax to advanced topics, empowering you to write efficient and high-performance code. Let’s Begin your Coding Journey….
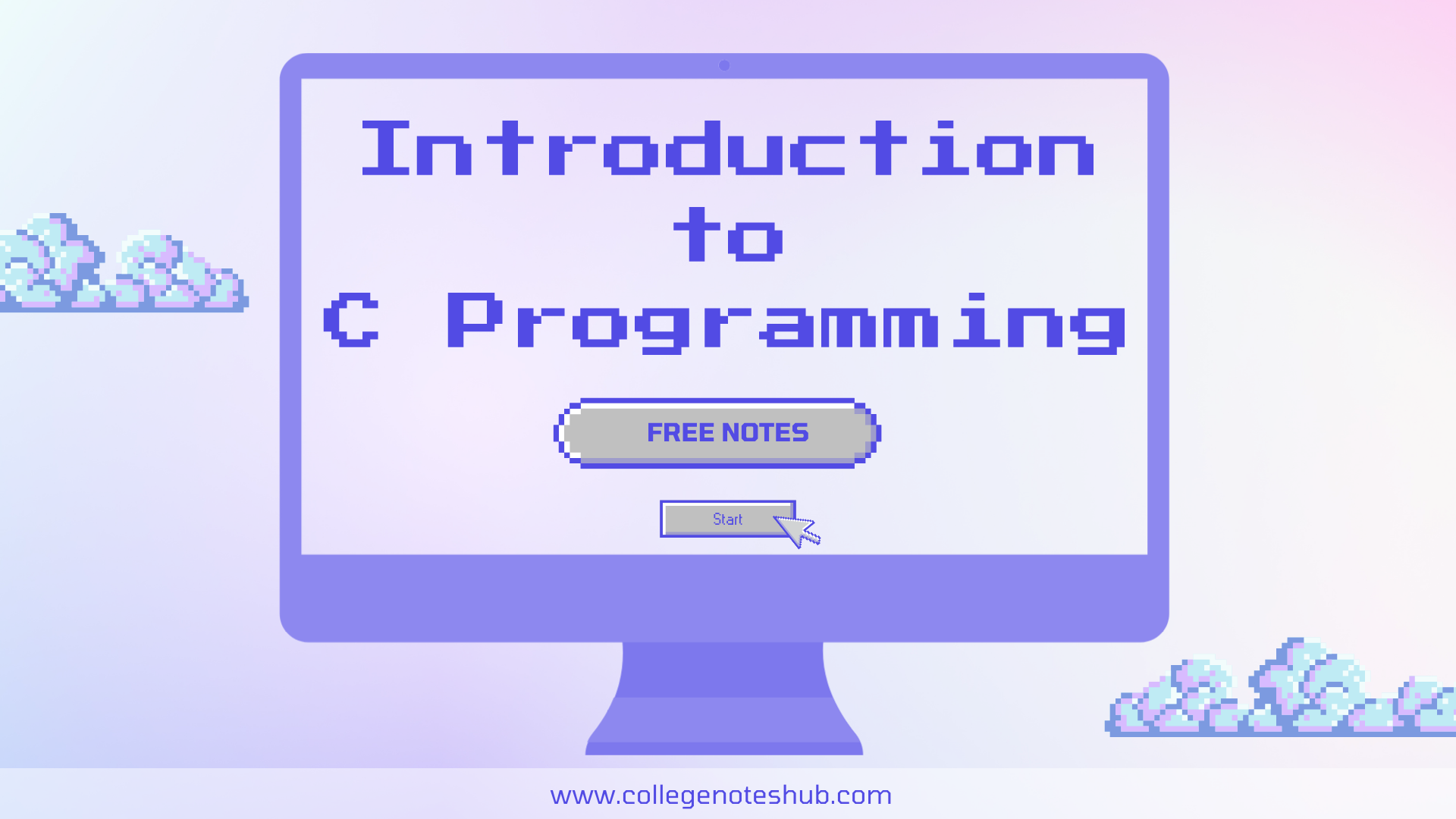
Table of Contents
What is C Programming?
C is a high-level, general-purpose programming language developed by Dennis Ritchie in 1972 at Bell Labs. It is widely used for developing system software, such as operating systems, compilers, and embedded systems. Despite its simplicity, it can handle complex operations and data structures efficiently.
Why Learn C Programming?
Learning C is essential for several reasons, especially if you want to build a strong foundation in programming. Here are some key benefits:
- Foundation for Modern Languages: C is the root of languages like C++, Java, and Python, making it easier to learn them after mastering C.
- High Efficiency: C offers direct access to system resources, ensuring high performance in system-level programming.
- Memory Control: With manual memory management through pointers, C teaches how computers handle data at the hardware level.
- Industry Standard: Used in operating systems, embedded systems, and more, C remains vital in software development.
- Portability: C code is highly portable, running across multiple platforms with minimal modifications.
- System Programming: Core system software, including operating systems and compilers, are written in C.
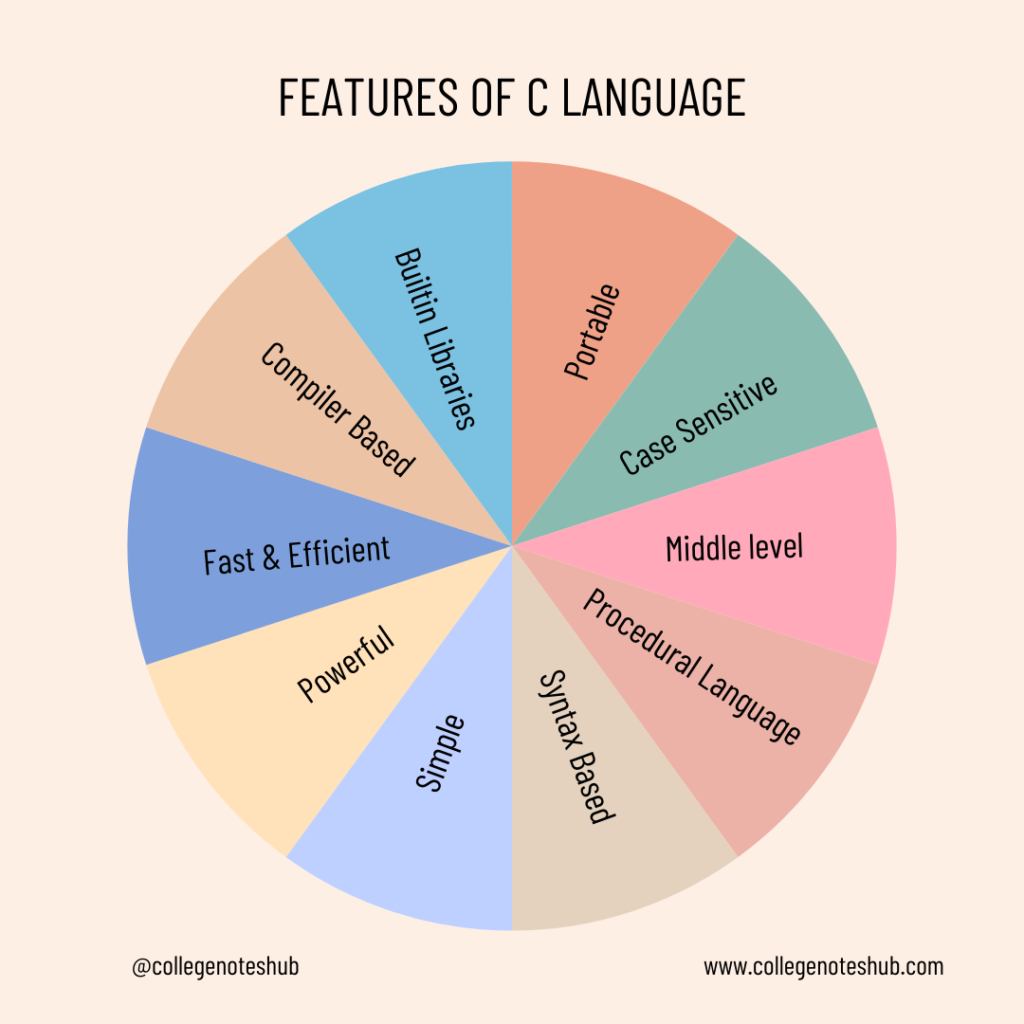
What We’re Going to Learn ?
Here is an navigation map through which you can easily click on the topic you want to learn :
- Introduction to C Programming
- History of C Programming
- Getting Started with C Programming
- C Language Syntax and Structure
- Variables in C
- Data Types in C
- What are Local & Global Variables
- Data Types and Variables in C
- Keywords in C
- Identifiers in C
- Operators in C
- Control Flow Statements in C
- Functions in C Programming
- Storage Classes in C
- Arrays in C
- Pointers in C
- Structures and Unions in C
- File Handling in C
- Preprocessor Directives in C
- Dynamic Memory Allocation in C
- Advanced Topics in C Programming
- Data Structures Implementation in C
- Best Practices for C Programming
- Standard Library Functions in C
- C Programming Projects and Case Studies
- C Programming Exam Preparation and Interview Questions
This includes all the overview on what we are going to learn in this C Programming Language Notes Series with you.
History of C Programming
Here is an brief explanation of History of C, We will discus about it’s History in next post :
- BCPL and B: The evolution of C began with languages like BCPL and B, which were used to write system software.
- Development of C: Dennis Ritchie, while working on the UNIX operating system at Bell Labs, developed C in the early 1970s as a more powerful alternative to B.
- The Rise of C: C gained popularity due to its performance and portability and was widely adopted for developing system software, including operating systems and compilers.
Structure of a C Program
The basic structure of a C program consists of several important elements:
#include <stdio.h> // Header file inclusion
int main() { // Main function
printf("Hello, World!"); // Output
return 0; // Return statement
}
CHere is the explaination of the above code :
- Header Files: Includes libraries like
stdio.h
, which allows you to use input/output functions likeprintf()
. - Main Function: This is the entry point of every C program, where execution begins.
- Statements: In the body of the
main()
function, we write the logic of the program. - Return Statement: Returns a value when the program terminates (usually 0).
Youtube Reference Links :
C programming is the backbone of computer science and a must-learn language for anyone looking to dive into software development. It provides you with a deep understanding of how software interacts with hardware and is still widely used in areas such as operating systems, embedded systems, and high-performance applications.
In the next post, We are going to discuss about the History of C Programming Language in detail. Stay tuned and be ready to code.